Document detection¶
The DocumentDetector provide functions to automatically detect and identify an ID document inside an image. The document type can be determined on the basis of pre-loaded document templates.
Important
The document detector requires the relevant AI models to run (see AI Models for details). It also requires the document templates to be loaded (see Document database for details).
- We provide 2 different models for document detection:
DocumentDetector2A : Best performance model
DocumentDetector2B : Lightweight model, but with stricter conditions : the 4 corners of the document must be clearly visible, and the total number of document templates among which to search must be reduced (max 2-3 documents)
Note
For both models, the document must be correctly oriented in the image. It is up to the user to rotate the image if necessary.
We provide 2 different functions for detection and/or identifying a document :
detect_document_by_name : Use when the document name is known, it will help and speed-up the process. In this case, the document name to pass is the full identifer, including the potential suffix _front or _back.
detect_document : Use when the type is unknown. A subset of document names or package names can be passed, otherwise the algorithm looks for every loaded document template.
Document Width Ratio¶
In order to speed-up the process, the DocumentDetector2A needs a a-priori information on the context of acquisition, namely the parameter document_width_ratio. This parameter should reflect the approximate proportion that the document (width-wise) occupies in the image, as shown in the example below. Note that the smaller this parameter, the slowest the detection. For landscape-like scenario, where this parameter is small, we strongly encourage you to provide a detectionZone to save some time.
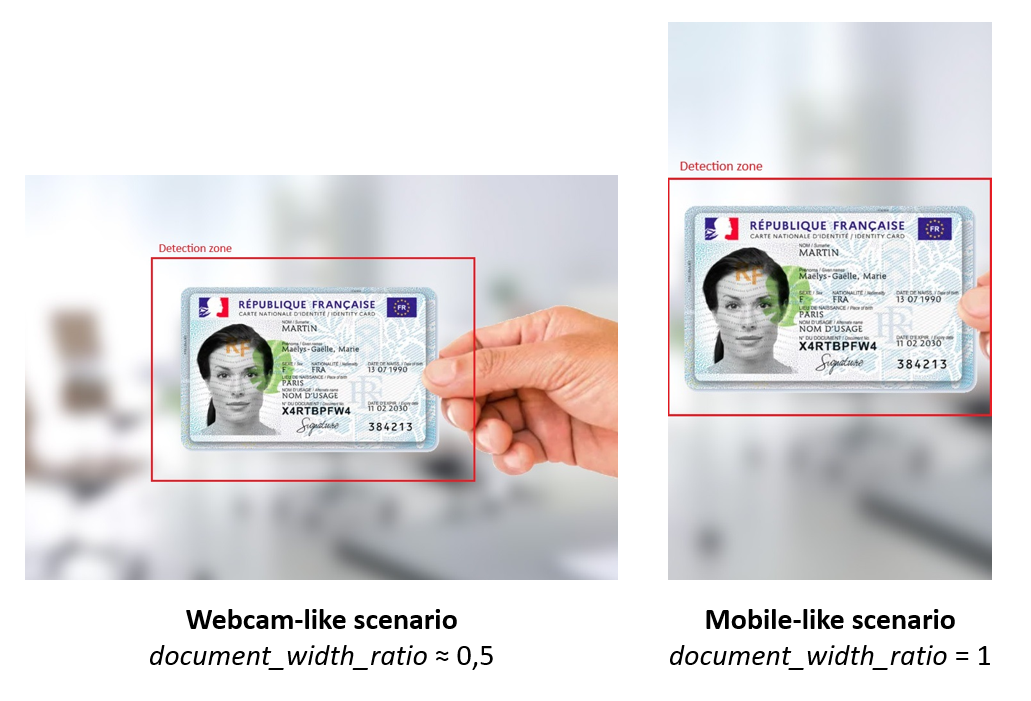
Detection zone¶
- The user can provide a detection zone to speed-up and improve the detection process.
For the DocumentDetector2A, the zone can be closely cropped around the expected position of the document (for example, in a real-time application, it could be its location in the previous frame)
For the DocumentDetector2B, the 4 corners of the document must be visible inside the zone.
Important
The output coordinates are kept in the referential of the full image.
Example¶
The example below demonstrates how to detect a document from an image.
DocumentLibrary.load_model(ai_models_path, DocumentModel.DOCUMENT_DETECTOR_2A, ProcessingUnit.CPU)
DocumentLibrary.load_document_template(os.path.join(document_template_path, "FRA_BO_03001_detector_2A_2.1.0.0.id3dr"))
# initialize the document detector
detector = DocumentDetector(
model = DocumentModel.DOCUMENT_DETECTOR_2A,
document_width_ratio = 1,
thread_count = 4
)
# load an image from a file.
image = DocumentImage.from_file(document_filename, PixelFormat.BGR_24_BITS)
# detect the document
detection_zone = Rectangle() # Empty detection zone = full image
document_templates_subset = StringList() # Empty subset = all loaded templates
document = detector.detect_document(image, detection_zone, document_templates_subset)
print(f"Confidence score: {document.confidence}")
if document.confidence >= detector.confidence_threshold:
# show document information
info = document.info
print(f"Document information:")
print(f"- Identifier : {info.identifier}")
print(f"- Name : {info.name}")
print(f"- Description : {info.description}")
print(f"- Category : {info.category.name}")
print(f"- Country : {info.country}")
print(f"- Format : {info.format.name}")
print(f"- Date : {info.date}")
print(f"- MRZ type : {info.mrz_type.name}")
print(f"- Page number : {info.page_number}")
image_crop = detector.align_document(image, document)
image_crop.to_file("crop.png", 0)
print(f"Document boundaries:")
for point in document.bounds:
print(f"- ({point.x}, {point.y})")
# align the document for OCR
aligned_image = detector.align_document(image, document)