Face detection¶
The FaceDetector class detects human faces in still images.
Important
Note
Call the FaceLibrary.loadModel Method to load the chosen model on application start.
Hint
On startup, call the FaceDetector.warmUp Method to prepare inference of the AI model.
Tolerance¶
The face detection tolerance depends on the AI model used. The following characteristics can be assumed:
Face angle tolerance |
yaw < ±45°, pitch < ±30°, roll < 45° |
Minimal interocular distance (IOD) |
10 pixels |
Warning
The algorithm searches for faces in the range [16px;512px]. If the image is too large to fit this range, one must resize it before the detection process.
Hint
For optimum facial recognition performance, a minimum distance of 100 pixels between eyes is recommended.
Detected face¶
The FaceDetector outputs a list of DetectedFace objects containing the following information:
Interocular distance (IOD)
Landmarks (eyes, nose and mouth)
Note
Only faces with a confidence score above the configured threshold are returned. See Parameters for details.
Face ID¶
The face ID is an auto-incremented value used to track faces.
Warning
The face ID is not linked to a user’s identity.
Confidence score¶
The confidence score ranges from 0 to 100. A high confidence score indicates that it is more likely that the face detected is really a face.
Face bounds¶
id3 Face SDK detects human faces and returns a rectangle around the detected faces, as shown in the figure below.
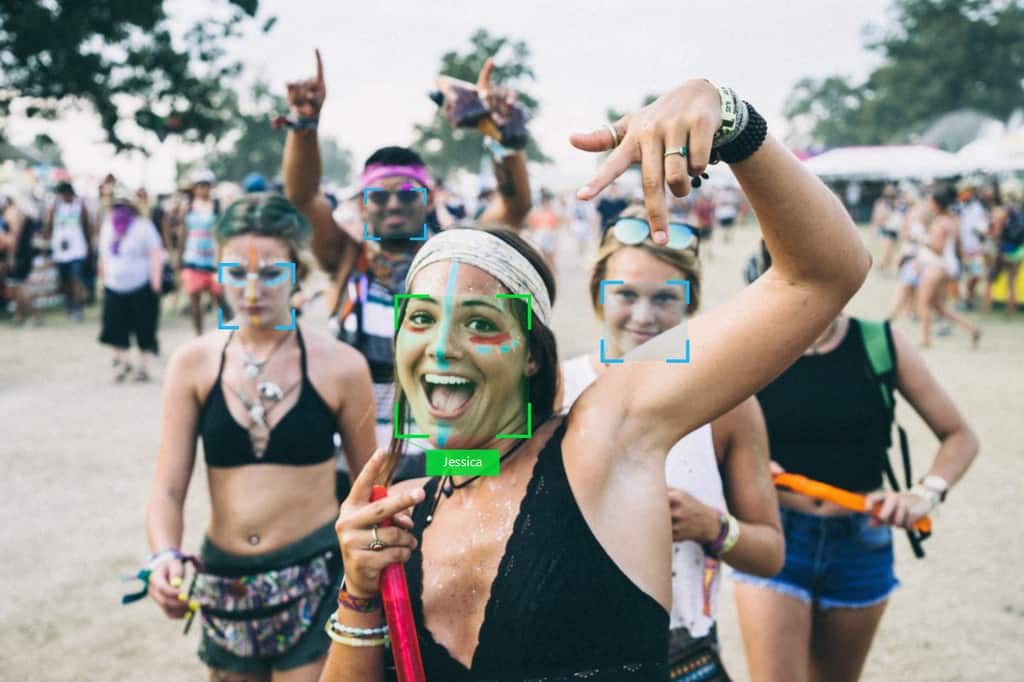
Portrait bounds¶
id3 Face SDK detects human faces and returns a rectangle for ISO/ICAO compliant portraits, as shown in the figure below.
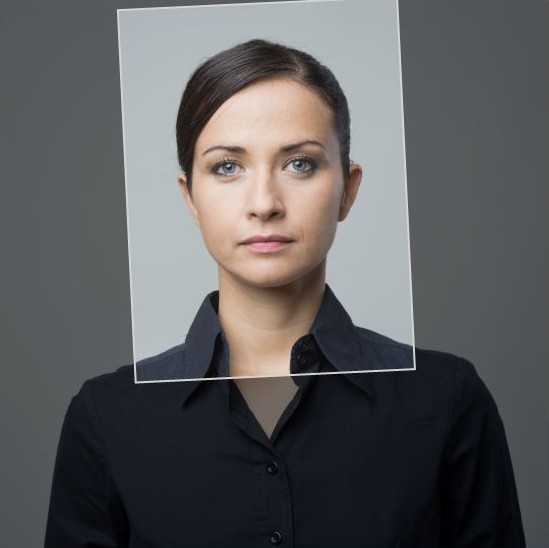
Parameters¶
The face detector is configured by default with the most common parameters. For specific applications, it may be useful to adjust certain parameters.
The following parameters are defined:
Name |
Description |
---|---|
Confidence threshold |
Value above which the detector considers the detected element to be a face.
Setting a high threshold reduces false detection but can increase the number of undetected faces.
|
Model |
Face detection models used to detect faces. |
NMS IoU threshold |
Non-maximum suppression (NMS) intersection-over-union (IoU) threshold.
Setting a high threshold allows to detect more overlapping faces which can be useful in a multi-face scenario. On the contrary, in a portrait scenario, a low NMS IoU threshold should be preferred.
![]() |
Thread count |
Number of threads to use for face detection. Default is 1. |
Example¶
The example below demonstrates how to detect faces in an image:
# create a new instance of the FaceDetector class.
face_detector = FaceDetector(
confidence_threshold = 50,
nms_iou_threshold = 40,
thread_count = 4
)
# warm up the face detector
face_detector.warm_up(512, 512)
# downscale image
downscaled_image = image.clone()
scale = downscaled_image.downscale(256)
# detect faces on the image.
detected_face_list = face_detector.detect_faces(downscaled_image)
# enumerate detected faces.
for face in detected_face_list:
bounds = face.bounds
# get the largest face
face = detected_face_list.get_largest_face()
# rescale detected face
face.rescale(1/scale)
# Dispose of all resources allocated to the FaceDetector.
del face_detector