One-to-One Comparison¶
Fingerprint comparison is the process of comparing a finger template against a previously stored reference template.
Since a finger template can contain 3 types of data (minutiae, minutia embeddings and/or finger embedding), the matching process adapts to the content of the reference and probe templates, and uses all data that is present in both the reference and probe templates.
Minutia-only matching can still be forced for interoperability purposes, if required.
Note
A FingerTemplateRecord should contain templates from a single user.
Threshold and decision¶
The decision is the result of the comparison between the biometric comparison similarity score of given biometric data and a predefined threshold to achieve the desired security level. If the score is greater than the predefined minimal matching score threshold, the biometric verification is successful.
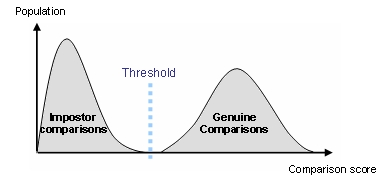
A decision threshold applied on the algorithm output score determines the operational FMR (False Match Rate), i.e. the probability of a system to falsely accept fingerprints (impostors). Since FMR and FNMR (False Non-Match Rate) are in inverse proportion to each other, FNMR will increase with higher decision thresholds.
Choosing the operational threshold is a trade-off between system security and user convenience. In one-to-one comparison mode, the operational threshold should be determined from the table below:
False Match Rate |
Decision threshold |
---|---|
1.0% |
2000 |
0.1% |
3000 |
0.01% |
4000 |
0.001% |
5000 |
0.0001% |
6000 |
0.00001% |
7000 |
0.000001% |
8000 |
0.000001% |
9000 |
0.0000001% |
10000 |
Performing a one-to-one comparison¶
The FingerMatcher module provides methods for comparing two finger templates or two finger template records. The methods return a comparison score, from 0 to 65535 representing the degree of similarity between the two templates.
Hint
To obtain a value between 0 and 100, we recommend applying the following formula to the matching score (S):
\(S' = MIN(100, S * 8 / 250)\)
Matching details¶
In the fingerprint comparison context it can be useful to know which minutiae were used by the matcher to output a “match” decision. Therefore id3 Finger SDK provides an optional component that contains the list of matched minutiae. See FingerMatcher.compareTemplatesInDetails Method for more information.
Example¶
The example below demonstrates how to compare two fingerprints:
ai_models_path = "C:/ProgramData/id3/models"
# initialize FingerExtractor
finger_extractor = FingerExtractor(
thread_count=4
)
# load an image from a file.
image1 = FingerImage.from_file("data/finger1_1.bmp", PixelFormat.GRAYSCALE_8_BITS)
image1.set_resolution(500)
# extract the template from the fingerprint image.
tic = time.perf_counter()
template1 = finger_extractor.create_template(image1)
toc = time.perf_counter()
print(f"Template 1 extracted in {1000*(toc-tic):0.1f} ms")
# load a second image from a file.
image2 = FingerImage.from_file("data/finger2_1.bmp", PixelFormat.GRAYSCALE_8_BITS)
image2.set_resolution(500)
# extract the template from the fingerprint image.
tic = time.perf_counter()
template2 = finger_extractor.create_template(image2)
toc = time.perf_counter()
print(f"Template 2 extracted in {1000*(toc-tic):0.1f} seconds")
# initialize a FingerMatcher
matcher = FingerMatcher()
# compare the templates
tic = time.perf_counter()
score = matcher.compare_templates(template1, template2)
toc = time.perf_counter()
print(f"Score = {score}")
print(f"Similarity = {min(100, score * 8 / 250):0.2f}%")
print(f"Match time = {1000*(toc-tic):0.3f} milliseconds")
# dispose all resources
del finger_extractor
del matcher
Comparison of 2 Template Records¶
The FingerMatcher module provides functions to compare template records, containing several templates for each user. These functions integrate advanced normalization process to ensure that the resulting score follows the score distribution and the thresholds shown above.
- These functions allow 2 comparison modes :
Trusted mode : The matches are performed only between corresponding position templates (and unknown positions)
Non trusted mode : All crossed matches are performed : for 10-fingers template records, a total of 100 matches are computed
Important
The template records must contain no more than 10 templates, with a single view for each position
Allowed positions are from 0 to 10 (included). Other positions in the template records are ignored !
Full sample of template records processing¶
FingerLibrary.load_model(MODELS_PATH, FingerModel.FINGER_MINUTIA_DETECTOR_4A, ProcessingUnit.CPU)
# ------- Load image records -----------
image_record_ref = FingerImageRecord.from_file(
FingerImageRecordFormat.AN2K_2011_TRANSACTION_TRADITIONAL_ENCODING, "data/an2k-type-04-tpcard.an2"
)
image_record_probe = FingerImageRecord.from_file(
FingerImageRecordFormat.AN2K_2011_TRANSACTION_TRADITIONAL_ENCODING, "data/an2k-type-14-tpcard.an2"
)
# ------- Extract all templates at once --------
extractor = FingerExtractor(minutia_detector_model=FingerModel.FINGER_MINUTIA_DETECTOR_4A, thread_count=4)
tpl_record_ref = extractor.create_template_record(image_record_ref)
tpl_record_probe = extractor.create_template_record(image_record_probe)
# --- Match template records ------
matcher = FingerMatcher()
# Scenario 1: Match only corresponding positions
match_score_trusted = matcher.compare_template_records(tpl_record_ref, tpl_record_probe, True)
print("Score with trusted positions :", match_score_trusted)
# Scenario 2: All cross-matches
match_score_non_trusted = matcher.compare_template_records(tpl_record_ref, tpl_record_probe, False)
print("Score with non trusted positions :", match_score_non_trusted)
# Scenario 3: Return detailed scores for each match, and no aggregated score.
match_details = matcher.compare_template_records_in_details(tpl_record_ref, tpl_record_probe, False)
print("Match details : ")
for _match in match_details:
position_ref = tpl_record_ref.get(_match.index_in_reference).position
position_probe = tpl_record_probe.get(_match.index_in_probe).position
print(" {} vs {} : {}".format(position_ref, position_probe, _match.score))