Feature Extraction¶
Feature extraction is the process of extracting unique features from a fingerprint image. It outputs a finger template to be used for one-to-one comparison or one-to-many search.
This component includes several complementary features:
Minutia detection – This is the core algorithm for fingerprint feature extraction. Minutiae are the most interoperable features and their encoding is precisely defined in ANSI and ISO standards.
Minutia encoding – Extracts a local signature for each minutia to improve overall matching performance.
Minutiae¶
Minutiae are points located at the places in the fingerprint image where friction ridges end or split into two ridges. Describing a fingerprint in terms of the location and direction of these ridge endings and bifurcations provides sufficient information to reliably determine whether two fingerprint records are from the same finger. A typical good-quality fingerprint image contains about 20-70 minutiae points; the actual number depends on the size of the sensor surface and how the user places his or her finger on the sensor. Each minutia has a “type” associated with it. There are two major types of minutiae:
Ridge endings
Ridge bifurcations
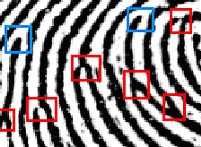
Minutia placement¶
To ensure the best interoperability with other systems, the minutia placement is as follow:
A minutia on a ridge ending is defined as the valley skeleton bifurcation point.
A minutia on a ridge bifurcation is defined as the ridge skeleton bifurcation point.
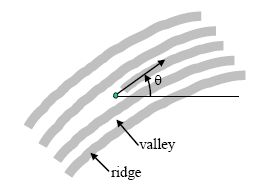
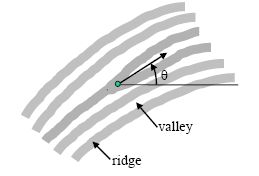
Minutia direction¶
The minutia angle is measured increasing counter-clockwise starting from the horizontal axis to the right. The angle granularity is 1.40625 (360/256) degrees.
Proprietary information¶
Minutia embedding¶
A minutia embedding is a local signature representing the neighboorhood of a minutia. It is generated using a deep learning model (see AI Models for details). It is complementary to the positions and angles of the minutiae described above.
Those signatures are stored in the proprietary section of a finger template at a cost of 16 bytes per minutia.
Finger Template¶
A finger template is a representation of a fingerprint using the fundamental notion of minutiae and optionally proprietary information. It is the result of the feature extraction process applied to a finger image.
A FingerTemplate object contains the following elements:
Minutia data
Proprietary fingerprint information (See minutia embeddings)
Image information
Height and width
Horizontal and vertical resolution
Impression type
Position of the finger
Image quality
Capture device information
Certification
Technology
Type identifier (vendor specific)
Vendor identifier (registered by IBIA)
It can be stored in a supported standard format for further usage. See Supported Formats for details.
Note
Several templates from one or more fingers can be grouped into a single FingerTemplateRecord Class.
Template quality¶
id3 Finger SDK computes a quality for each created template. This value between 0 and 100 aims at predicting the ability of a template to perform well at matching. A very high template quality means that error rates during verification will be as low as possible. On the contrary, a low template quality will induce errors during verification and more precisely false rejections: a genuine person will possibly be rejected by the matching algorithm because of the poor quality of the presented template. This is why it is important to check template quality in an enrollment process.
Example¶
The example below demonstrates how to generate a FingerTemplate from a FingerImage:
# initialize FingerExtractor
finger_extractor = FingerExtractor(
thread_count=4
)
# load an image from a file.
image = FingerImage.from_file("data/finger1_1.bmp", PixelFormat.GRAYSCALE_8_BITS)
image.set_resolution(500)
# extract the template from the fingerprint image.
tic = time.perf_counter()
finger_template = finger_extractor.create_template(image)
toc = time.perf_counter()
print(f"Template extracted in {1000*(toc-tic):0.1f} ms")
print(f"Template quality: {finger_template.quality}")
# export the finger template to a buffer in ISO/IEC 19794-2:2011 format.
template_data = finger_template.to_buffer(FingerTemplateFormat.ISO19794_2_2011)
print(" ".join("{:02x}".format(x) for x in template_data))
# dispose all resources
del finger_extractor